Core Widgets¶
We refer to the widgets that are bundled with Jibe as the Core Widgets. This is a minimal collection of widgets that allow you to compose the great majority of more complex widgets, simply by combining them together.
Contents:
Widget¶
All other widgets inherit from the Widget class. By itself it is of little use, but it serves as a blank canvas to build other widgets from scratch, at a low level. For most applications you will not need to use this class directly, instead, you will inherit from other widgets and will customize their behavior. See the Widget API for more details.
Div¶
This is identical to Widget. It represents a <div> … </div>
in the DOM (a plain empty container in your page).
Button¶
A button. Represents a <button> … </button>
in the DOM.
It has 1 property: label, which is displayed on the face of
the button, and triggers 1 event: click.
btn = Button('button')
btn.register('click', click_handler)
Input¶
A single-line text input. It represents an <input>
in the DOM.
It has 1 property: value, and triggers 1 event: change.
input = Input('input')
input.register('change', change_handler)
Label¶
A simple text label. It has 1 property: value, and no events.
It represents a <div><p> ... </p></div>
structure in the DOM.
label = Label('I am a label')
CheckBox¶
A check box. It represents a <input type="checkbox"/>
in the DOM.
It has one property: checked and triggers one event: change.
chk = CheckBox()
chk.register('change', on_change)
Dropdown¶
A selection drop-down. It represents a <select> ... </select>
in the DOM.
It has two properties: value and options, and triggers one event: change.
dropdown = Dropdown(
options=[
'apples',
'banannas',
'chocolate'
]
)
dropdown.register('change', on_change)
SelectMultiple¶
A multiple-selection list. It represents a
<select multiple="multiple"> ... </select>
in the DOM.
It has two properties: value and options.
sel = SelectMultiple(
options=[
'apples',
'banannas',
'chocolate'
]
)
sel.register('change', on_change)
ProgressBar¶
Simple horizontal progress bar. It has one property: value, which
represents the percentage of completion (0 to 100). This is a composite
widget (a Div
of fixed size for the background and another Div
with variable width for the progress). It is not a “core” widget, since
you can build it yourself from other core widgets, but it is provided
as a example.
bar = ProgressBar(30)
Image¶
An image/png. It represents a <img/>
in the DOM and has one
property: data. The data is the image data, as a base64-encoded utf8 string
(see the code below).
import base64
with open("image.png", "rb") as img:
data = base64.encodebytes(img.read()).decode('utf8')
img = Image()
img.data = data
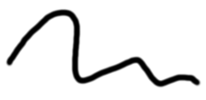
Redirect¶
An invisible widget that allows redirecting the browser to a different url.
redir = Redirect()
redir.redirect('/other')